Transaction
#
Send to an addressYou can delegate Marina to create, fund, blind, sign and broadcast a Liquid transaction to a recipient. You do not need to know anything about current balance, although is suggested to retrieve and display the balances in your application for better UX and to check beforehand for sufficient funds.
This will prompt the user to allow blinding & signing a transaction.
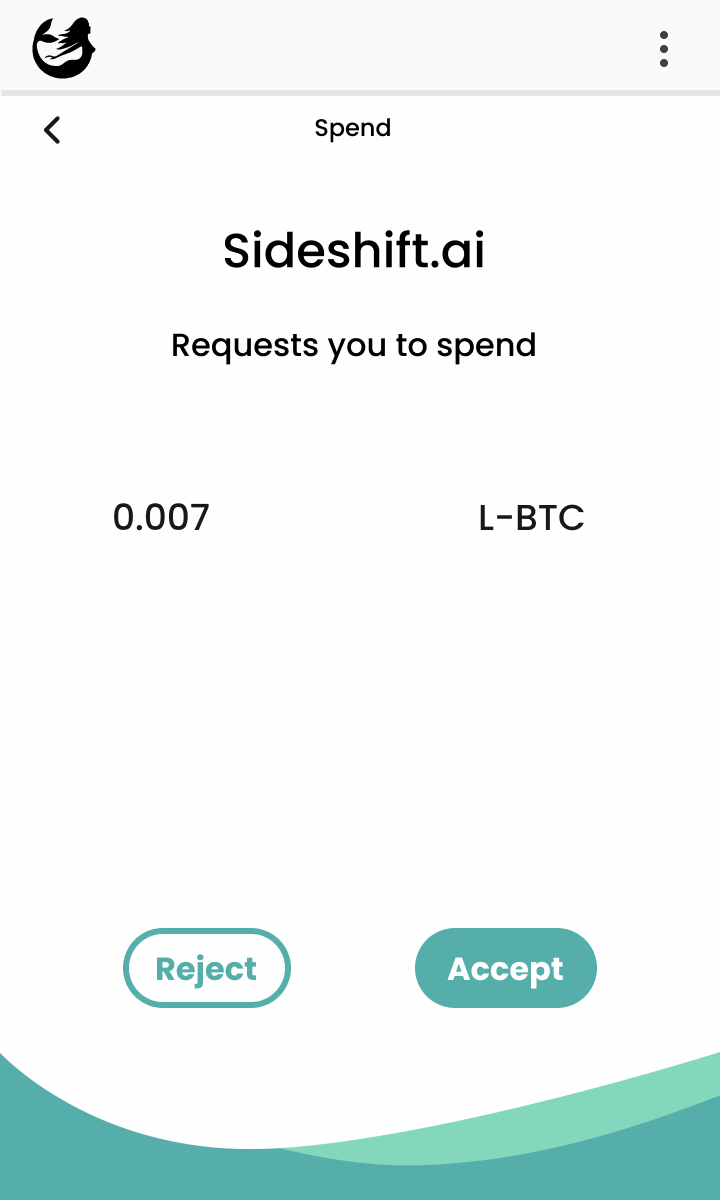
If the prompt is accepted, the transaction will be blinded, signed and broadcasted.
#
Custom transactionDevelopers can build custom transactions using the unspents of the exposed addresses and can delegate Marina to sign with the user consent.
This will prompt the user to allow signing the custom transaction
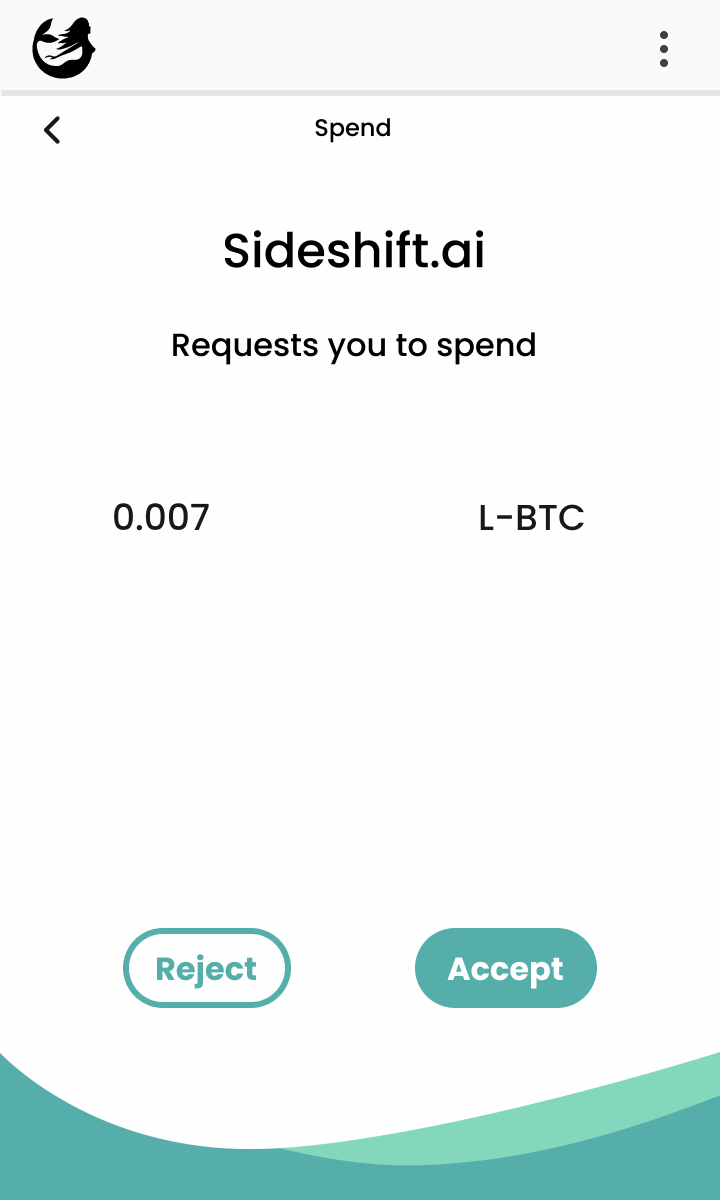
If the prompt is accepted, will be possible to retrieve the signed base64 transaction